REST API
- GET API
- 리소스 취득
- Annotation
- @GetMapping : Get Resource 설정
- @RequestParam : URL Query Param Parsing
- @PathVariable : URL Path Variable Parsing
- POST API
- 리소스 생성, 추가
- Annotation
- @PostMapping : Post Resource 설정
- @RequestBody : Request Body 부분 파싱
- @PathVariable : URL Path Variable 파싱
- @JsonProperty : json naming
- @JsonNaming : class json naming
- PUT API
- 리소스 갱신, 생성
- Annotation
- @PutMapping : Put Resource 설정)
- @RequestBody : Request Body 부분 파싱
- @PathVariable : URL Path Variable 파싱
- DELETE API
- 리소스 삭제
- Annotation
- @DeleteMapping : Delete Resource 설정
- @RequestParam : URL Query Param 파싱
- @PathVariable : URL Path Variable 파싱
- Object : Query Param Object로 파싱
- @ResponseBody 문자 반환
@Controller
public class HelloController {
@GetMapping("hello-string")
@ResponseBody
public String helloString(@RequestParam("name") String name) {
return "hello " + name;
}
}
- @ResponseBody 객체 반환
@Controller
public class HelloController {
@GetMapping("hello-api")
@ResponseBody
public Hello helloApi(@RequestParam("name") String name) {
Hello hello = new Hello();
hello.setName(name);
return hello;
}
static class Hello {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
}
- ResponseBody 사용 원리(출처 : 인프런 스프링 입문 - 코드로 배우는 스프링 부트, 웹 MVC, DB 접근 기술)
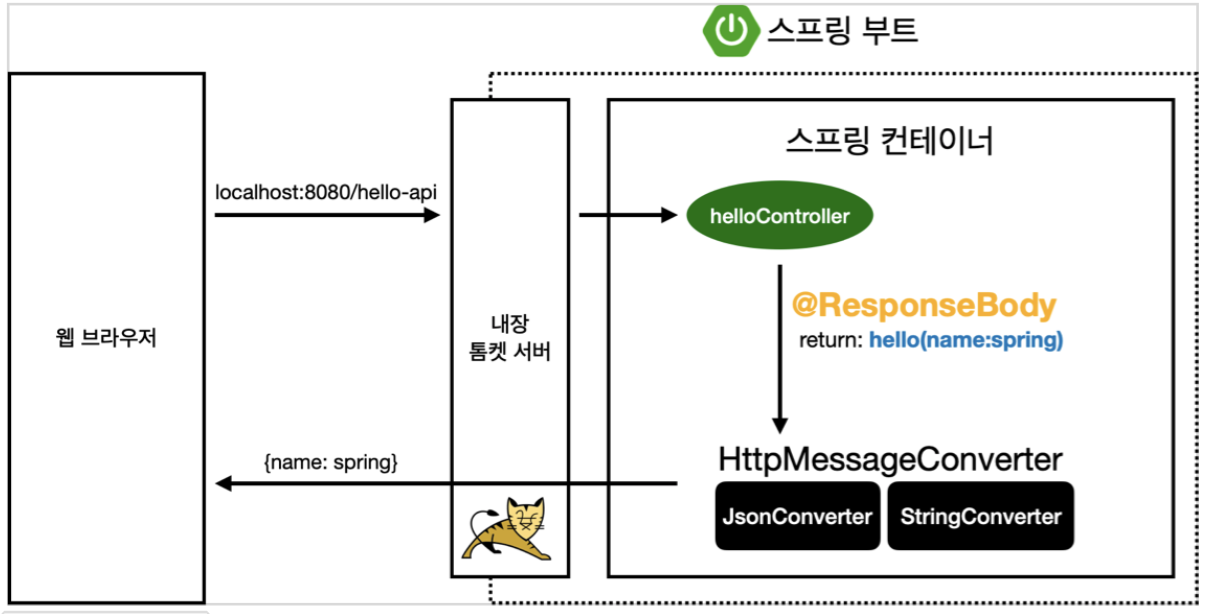